Thursday, 25 April 2019
Add Arduino Uno Library into Proteus software
Monday, 22 April 2019
Thursday, 18 April 2019
TELNET using Cisco Packet Tracer
TELNET using Cisco Packet Tracer
Monday, 8 April 2019
CP : Reverse Order using Pointer
Write a program using a pointer to read in an array of integers and print its elements in reverse order.
Code :
#include<stdio.h>
#include<conio.h>
void main()
{
int i, arr[30];
int *ptr;
ptr = &arr[0];
printf("\nEnter 5 integers into array: \n");
for (i = 0; i < 5; i++)
{
scanf("%d", ptr);
ptr++;
}
ptr = &arr[4];
printf("\nElements of array in reverse order are :\n");
for (i = 5; i >0; i--)
{
printf("\n %d ", i, *ptr);
ptr--;
}
getch();
}
Output:
CP : Union in C
Union in C
Unions are conceptually similar to structures. The syntax to declare/define a union is also similar to that of a structure.
The only differences are in terms of storage. In structure each member has its own storage location, whereas all members of union uses a single shared memory location which is equal to the size of its largest data member.
This implies that although a union may contain many members of different types, it cannot handle all the members at the same time. A union is declared using the
union
keyword.Structure vs. Union
CP : Structure with example
C Structure is a collection of different data types which are grouped together and each element in a C structure is called member.
- If you want to access structure members in C, the structure variable should be declared.
- Many structure variables can be declared for the same structure and memory will be allocated for each separately.
- It is a best practice to initialize a structure to null while declaring if we don’t assign any values to structure members.
DIFFERENCE BETWEEN C VARIABLE, C ARRAY, AND C STRUCTURE:
- A normal C variable can hold only one data of one data type at a time.
- An array can hold a group of data of the same data type.
- A structure can hold a group of data of different data types and Data types can be int, char, float, double and long double, etc.
Example :
#include <stdio.h>
struct student
{
char name[50];
int roll;
float marks;
} s;
int main()
{
printf("Enter information:\n");
printf("Enter name: ");
scanf("%s", &s.name);
printf("Enter roll number: ");
scanf("%d", &s.roll);
printf("Enter marks: ");
scanf("%f", &s.marks);
printf("Displaying Information:\n");
printf("Name: ");
puts(s.name);
printf("Roll number: %d\n",s.roll);
printf("Marks: %.1f\n", s.marks);
return 0;
}
Output :
CP : Structures
Why Use Structures?
And here is the sample run...
Enter names, prices and no. of pages of 3 books
A 100.00 354
C 256.50 682
F 233.70 512
And this is what you entered
A 100.000000 354
C 256.500000 682
F 233.700000 512
A structure contains a number of data types grouped together. These data types may or may not be of the same type. The following example illustrates the use of this data type.
main( )
{
struct book
{
char name ;
float price ;
int pages ;
} ;
struct book b1, b2, b3 ;
printf ( "\nEnter names, prices & no. of pages of 3 books\n" ) ;
scanf ( "%c %f %d", &b1.name, &b1.price, &b1.pages ) ;
scanf ( "%c %f %d", &b2.name, &b2.price, &b2.pages ) ;
scanf ( "%c %f %d", &b3.name, &b3.price, &b3.pages ) ;
printf ( "\nAnd this is what you entered" ) ;
printf ( "\n%c %f %d", b1.name, b1.price, b1.pages ) ;
printf ( "\n%c %f %d", b2.name, b2.price, b2.pages ) ;
printf ( "\n%c %f %d", b3.name, b3.price, b3.pages ) ;
}
And here is the output...
Enter names, prices and no. of pages of 3 books
A 100.00 354
C 256.50 682
F 233.70 512
And this is what you entered
A 100.000000 354
C 256.500000 682
F 233.700000 512
Declaring a Structure
struct <structure name>
{
structure element 1 ;
structure element 2 ;
structure element 3 ;
......
......
} ;
Once the new structure data type has been defined one or more variables can be declared to be of that type. For example, the variables b1, b2, b3 can be declared to be of the type struct book, as, struct book b1, b2, b3 ;
Note the following points while declaring a structure type:
(a) The closing brace in the structure type declaration must be followed by a semicolon.
(b) It is important to understand that a structure type declaration does not tell the compiler to reserve any space in memory. All a structure declaration does is, it defines the ‘form’ of the structure.
(c) Usually, the structure type declaration appears at the top of the source code file, before any variables or functions are defined. In very large programs they are usually put in a separate header file, and the file is included (using the preprocessor directive #include) in whichever program we want to use this structure type.
We have seen earlier how ordinary variables can hold one piece of information and how arrays can hold a number of pieces of information of the same data type. These two data types can handle a great variety of situations. But quite often we deal with entities that are a collection of dissimilar data types.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and a number of pages in it (an int). If data about say 3 such books is to
be stored, then we can follow two approaches:
(a) Construct individual arrays, one for storing names, another for storing prices and still another for storing a number of pages.
(b) Use a structure variable.
Let us examine these two approaches one by one. For the sake of programming, convenience assumes that the names of books would be single character long. Let us begin with a program that uses arrays.
{
char name[3] ;
float price[3] ;
int pages[3], i ;
printf ( "\nEnter names, prices and no. of pages of 3 books\n" ) ;
for ( i = 0 ; i <= 2 ; i++ )
scanf ( "%c %f %d", &name[i], &price[i], &pages[i] );
printf ( "\nAnd this is what you entered\n" ) ;
for ( i = 0 ; i <= 2 ; i++ )
printf ( "%c %f %d\n", name[i], price[i], pages[i] );
}
And here is the sample run...
Enter names, prices and no. of pages of 3 books
A 100.00 354
C 256.50 682
F 233.70 512
And this is what you entered
A 100.000000 354
C 256.500000 682
F 233.700000 512
A structure contains a number of data types grouped together. These data types may or may not be of the same type. The following example illustrates the use of this data type.
main( )
{
struct book
{
char name ;
float price ;
int pages ;
} ;
struct book b1, b2, b3 ;
printf ( "\nEnter names, prices & no. of pages of 3 books\n" ) ;
scanf ( "%c %f %d", &b1.name, &b1.price, &b1.pages ) ;
scanf ( "%c %f %d", &b2.name, &b2.price, &b2.pages ) ;
scanf ( "%c %f %d", &b3.name, &b3.price, &b3.pages ) ;
printf ( "\nAnd this is what you entered" ) ;
printf ( "\n%c %f %d", b1.name, b1.price, b1.pages ) ;
printf ( "\n%c %f %d", b2.name, b2.price, b2.pages ) ;
printf ( "\n%c %f %d", b3.name, b3.price, b3.pages ) ;
}
And here is the output...
Enter names, prices and no. of pages of 3 books
A 100.00 354
C 256.50 682
F 233.70 512
And this is what you entered
A 100.000000 354
C 256.500000 682
F 233.700000 512
Declaring a Structure
struct <structure name>
{
structure element 1 ;
structure element 2 ;
structure element 3 ;
......
......
} ;
Once the new structure data type has been defined one or more variables can be declared to be of that type. For example, the variables b1, b2, b3 can be declared to be of the type struct book, as, struct book b1, b2, b3 ;
Note the following points while declaring a structure type:
(a) The closing brace in the structure type declaration must be followed by a semicolon.
(b) It is important to understand that a structure type declaration does not tell the compiler to reserve any space in memory. All a structure declaration does is, it defines the ‘form’ of the structure.
(c) Usually, the structure type declaration appears at the top of the source code file, before any variables or functions are defined. In very large programs they are usually put in a separate header file, and the file is included (using the preprocessor directive #include) in whichever program we want to use this structure type.
Friday, 5 April 2019
How to increase by-default track-width in Proteus 8
Here you will learn how to increase track width. If you are going to do etching at home, you need to increase track width. By-default track width is very small, so it is helpful if you increase by-default trackwidth.
Click Here
RIP Routing Implementation and Verification using Cisco Packet Tracer
Here you can learn the implementation of RIP Routing using Cisco Packet Tracer. Here How to verify your RIP topology that also has been explained.
Click Here
Tuesday, 2 April 2019
CP : Pointer explanation
What are the Pointers?
A pointer is a variable whose value is the address of another variable, i.e., direct address of the memory location.
The general form of a pointer variable declaration is −
type *var-name;
type is the pointer's base type
var-name is the name of the pointer variable
asterisk * used to declare a pointer is the same asterisk used for multiplication.
Take a look at some of the valid pointer declarations −
int *ip; /* pointer to an integer */ double *dp; /* pointer to a double */ float *fp; /* pointer to a float */ char *ch /* pointer to a character */
How to Use Pointers?
There are a few important operations, which we will do with the help of pointers very frequently.
(a) We define a pointer variable,
(b) assign the address of a variable to a pointer and
(c) finally access the value at the address available in the pointer variable.
This is done by using unary operator * that returns the value of the variable located at the address specified by its operand. The following example makes use of these operations −
EXAMPLE:
#include <stdio.h>
int main ()
{
int var = 20; /* actual variable declaration */
int *ip; /* pointer variable declaration */
ip = &var; /* store address of var in pointer variable*/
printf("Address of var variable: %x\n", &var );
/* address stored in pointer variable */
printf("Address stored in ip variable: %x\n", ip );
/* access the value using the pointer */
printf("Value of *ip variable: %d\n", *ip );
return 0;
}
Reference operator (&) and Dereference operator (*)
"
&
"is calledthe reference operator. It gives you the address of a variable.
Likewise, there is another operator that gets you the value from the address, it is called a dereference operator "
*
."
Explanation
Proteus : How to Increase Track Width ( individually and default both )
Dear
Here I have explained how you can increase the default track width. If you are doing the etching process at your home, you need a thick track width.
Watch this video for a detailed explanation.
Click Here
Here I have explained how you can increase the default track width. If you are doing the etching process at your home, you need a thick track width.
Watch this video for a detailed explanation.
Click Here
Monday, 1 April 2019
Networking: Assign IP using CLI and GUI
Dear all
Here I have explained two methods of assigning IP- Address in Router
1: using CLI ( Command Line- Interface )
2: Using GUI ( Graphical User-Interface )
You can watch this video
Click Here
Here I have explained two methods of assigning IP- Address in Router
1: using CLI ( Command Line- Interface )
2: Using GUI ( Graphical User-Interface )
You can watch this video
Click Here
Subscribe to:
Posts (Atom)
LAB 7 Arduino with Seven Segment Display || Arduino Tutorial || Code and Circuit Diagram || Project
LAB 7 Arduino with Seven Segment Display || Arduino Tutorial || Code and Circuit Diagram || Project Dear All We will learn how to Connec...
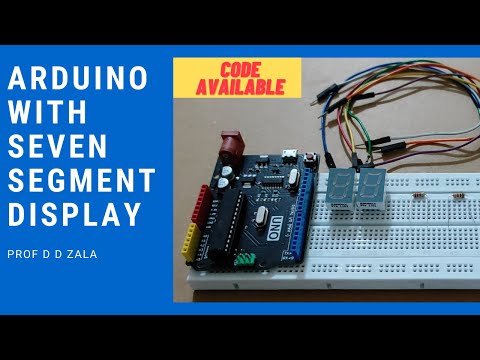
-
Dear Here I have explained how you can increase the default track width. If you are doing the etching process at your home, you need a ...
-
Here you will learn how to increase track width. If you are going to do etching at home, you need to increase track width. By-default trac...
-
Question: What do you mean by error detecting codes? Explain Two-dimensional Parity check. Answer Error: A condition when t...